
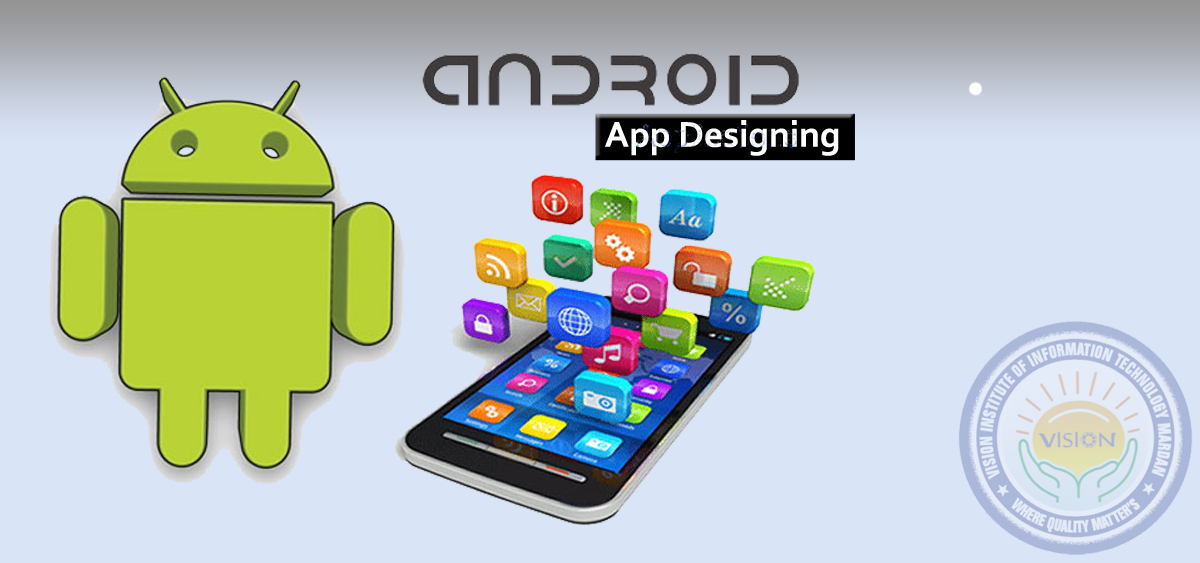

Instructors
Mr. Saif Ullah
Timing
Morning, Afternoon
Duration
3 Months
Fee
PKR 50,000
Contact
+92-331-5875434
Brief introduction
Android Development is the process of creating Android apps. This course is designed for students who are new to programming, and want to learn how to develop Android apps.
By the end of this course, student have all the skills required to design and develop own Android based mobile apps or even start a career with one of the thousands of companies which working in Android app designing.
java Introduction
- What You Should Already Know
- What is Java?
- History of Java
- Java Programming structure
- JVM
- JDK
- JRE
Environment Setup
- Java Installation
- Difference Between Path and Classpath
- How to Compile a Java File Using javac
- Run Our First Program Using Java
Java Basic Variable and Data types
- Java Variables
- Java Data Types
- Unicode System
- Java Operators
- Java Keywords
Java Control Statements
- Java If-else
- Java switch
- Java For Loop
- Java While Loop
- Java Do While Loop
- Java Break
- Java Continue
- Java Comments
Miscellaneous
- Java Strings Class
- Java Date & Time
- Java Methods
- Java Inner classes
Java Arrays, Matrix, Methods and Exception Handling
- Arrays
- Matrix
- Methods
- Exception Handling
Java Object Oriented Programming (OOPS) (1)
- Introduction
- Overview
- Classes & Objects
- Methods
- Polymorphism
- Constructors
- [static] keyword
- [this] keyword
Java Object Oriented Programming (OOPS) (2)
- Inheritance
- Encapsulation
- Abstraction
- [abstract] keyword
- Interfaces
Java Collection Framework (1)
- Overview
- Collection Interface
- Array List
- Iterator
- Stack
- Queue and LinkedList
- Priority Queue
- Array Deque
- HashSet
- Linked HashSet
Java Collection Framework (2)
- Tree Set
- Sets of Custom Classes (hash Code and equals)
- HashMap
- Tree Map
- Arrays Class
- Collections Class
- Sorting Custom Collections
- Comparable Interface
- Comparator Interface
Kotlin Programming Language (1)
- Introduction
- Kotlin Playground
- String Templates
- var and val
- Nullable Types
- if-else and when
- Arrays
Kotlin Programming Language (2)
- Loops
- Ranges
- Collection Framework
- Functions
- Higher-order functions
- OOPS
- filter and map
- scope function (let, run)
Android Studio Installation and Overview and Creating our First App
- Install android studio
- Install command line tools with sdk
- Install emulator
- Running app on emulator
- Running app on physical Device
- Create our First App
Activities in Android
- What are Activities in Android?
- How Activities work in android?
Layouts in Android XML
- Frame Layout
- Linear Layout
- Relative Layout
- Constraint Layout
View Elements in Android XML
- Text View
- Button
- Edit Text
- Image View
- Video View
Writing User Interface Code and making an App
- On Click Listener
- On Long Click Listener
- Currency converter app (Project)
Android Widget (1)
- UI Widgets
- Working with Button
- Toast
- Custom Toast
- Toggle Button
- Check Box
- Custom Check Box
- Radio Button
- Dynamic Radio Button
- Custom Radio Button
- Alert Dialog
- Spinner
- Auto Complete Text View
- Rating Bar
Android Widget (2)
- WebView
- Seek Bar
- Date Picker
- Time Picker
- Analog and Digital
- Progress Bar
- Vertical Scroll View
- Horizontal Scroll View
- Image Switcher
- Image Slider
- View Stub
- Tab Layout
- Tab Layout with Frame Layout
- Search View
- Search View on Toolbar
- Edit Text with Text Watcher
Intents in Android
- IMPLICIT INTENT
- EXPLICIT INTENT
FRAGMENTS in Android (1)
- LIST VIEW
- Custom List View
- RECYCLER VIEW
- DIALOUGE'S
FRAGMENTS in Android (2)
- BUTTOM SHEET (MATIRIAL DESIGN)
- NAVIGATION DRAWER
- VIEW PAGER'S (CLICK TO GO TO NEXT PAGE LIKE PROFILE, HOME ETC)
- TAB'S
Android Networks
- COMPLEX UI
- HTTP CLIENT
- a) GET REQUEST
- b) POST REQUEST
LIBRARIE'S
- OK HTTP
- a) IMAGE DOWNLOAD
- b) NORMAL HTTP REQUEST
- RETROFIT
- a) INTRACT TO REST API'S
- APPLO ANDRIOD
- a) GRAPH QL
- 4) GLIDE
- a) IMAGE LOADING FROM INTERNET
- VOLLEY
- a) GET REQUEST
- b) POST REQUEST
- Picasso
- a) GET REQUEST
- b) POST REQUEST
Let's Make Some Interesting Project using our knowledge
- Medium clone App (Blogging Site) (visit to realworld.io) (Project)
- Instagram Story Clone App (Using IMGUR API) (Project)
- Daily notes App / Daily Diary App (Project)
- Shopping List App (Project)
Accessing Some data From Storage in Android
- COMPLEX UI
- HTTP CLIENT
- a) GET REQUEST
- b) POST REQUEST
Accessing Some data From Storage in Android
- Playing Audio
- Video View
- Camera (Take photo, Record Video)
DEPLOYEMENT/ PRODUCTION OR FIREBASE
- Intro to firebase
- Connect App with Firebase
- User Auth
- Firebase Real time database
- Firebase Storage
- Firebase Cloud database
- Upload Image
- Upload Video
- Sign In with Google
- Sign in With Facebook
- Sign in with email
- Sign in with phone
- Firebase With Recycler view
Let’s make an interesting App using Firebase
- A Quiz App (Project)
- A social Media App (Like Facebook, Instagram) (Project)
Work Manager
- Background Task
JOB SCHEDULAR
- Used in Older Android
Saving Data (Databases)
- Shared preferences
- SQL Lite Database
- Room Database
Camera X
- Now Used in Android
Google Map
- How to implement google map
- How to add overlays in google map
- How to use Geo coder class in android
Let's Do Some more Projects
- News app using news API (Project)
- Weather App Using weather API (Project)
- Note Taking App (Project)
- Audio Player App (Project)